In this article, we will explain how to get all form values at once using Vanilla JavaScript.
This method can be useful when sending form data to the server using ajax or fetch, or when performing client-side validation with JavaScript before sending it to the server.
Table of Contents
- FormData class
- How to use the FormData class
- Inspecting the contents of a FormData object
- Getting values from the FormData object with key specifications
- Setting values in the FormData object with key specifications.
- Removing a key and its value from the FormData object with key specifications
- Looping through the FormData object.
- Sending the FormData object to the server-side.
FormData class
JavaScript provides the FormData class, which allows you to get all form values without the need for any special libraries.
If you are using jQuery, you can use the serialize method or serializeArray method to achieve a similar result. However, when you want to get all form values at once using Vanilla JavaScript, you can utilize the FormData class.
How to use the FormData class
Let’s now look at how to use the FormData class.
First, let’s assume we have the following HTML structure.
(*We will proceed with the assumption that we’ll be getting form values from this HTML.)
<form>
<input type="text" name="name" value="Jonny">
<input type="radio" name="gender" value="man" checked>Man
<input type="radio" name="gender" value="Woman">Woman
<input type="checkbox" name="language[]" value="php" checked>php
<input type="checkbox" name="language[]" value="java" checked>java
<input type="checkbox" name="language[]" value="python">python
<input type="submit" value="SUBMIT">
</form>
The JavaScript code to get all form values using the FormData class is as follows.
By using new FormData(formElement), a FormData object containing the form values is generated.
The e.preventDefault() prevents the form from being submitted to the server directly when the “Submit” button is clicked.
document.querySelector('form').addEventListener('submit', function(e){
const formData = new FormData(this)
e.preventDefault()
})
Inspecting the contents of a FormData object
When outputting the retrieved form values using console.log to verify if they are being correctly obtained, you would notice something: the form values seem to be nowhere to be found
document.querySelector('form').addEventListener('submit', function(e){
const formData = new FormData(this)
console.log(formData) // Incorrect way to check form values
e.preventDefault()
})
When you directly console.log the FormData object, you can’t see the form values.
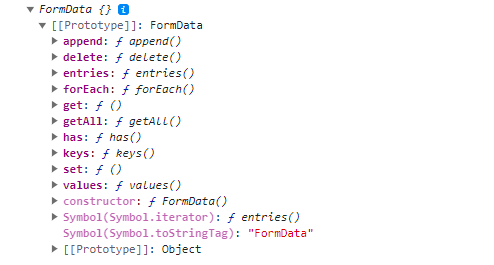
To verify if you have successfully got the form values using FormData, you can use the spread operator to expand them into an empty array as shown below.
document.querySelector('form').addEventListener('submit', function(e){
const formData = new FormData(this)
console.log([...formData]) // Correct way to check form values
e.preventDefault()
})
By using the spread operator to expand the FormData into an empty array, you can inspect the form values.
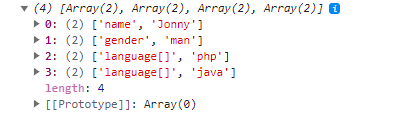
Getting values from the FormData object with key specifications
The FormData class provides the get method and the getAll method, which allow you to get valueswith key specifications.
method | response type | purpose | if the key does not exist |
---|---|---|---|
get | TEXT type | Getting a single value | Null is returned |
getAll | Array type | Getting multiple values | Empty array is returned |
document.querySelector('form').addEventListener('submit', function(e){
const formData = new FormData(this)
console.log(formData.get('name')) // get method because it is single value
console.log(formData.get('gender')) // get method because it is single value
console.log(formData.getAll('language[]')) // getAll method because it is multiple values
e.preventDefault()
})
get method can get single value, getAll method can get multiple values.

Setting values in the FormData object with key specifications.
The FormData class provides the set method, which allows you to set values in the FormData object with key specifications.
You can set a value using set(‘key’, ‘value’).
document.querySelector('form').addEventListener('submit', function(e){
const formData = new FormData(this)
formData.set('age', '30') // set method allows you to set values in the FormData object
console.log([...formData])
e.preventDefault()
})
set method allows you to set value in the FormData object.
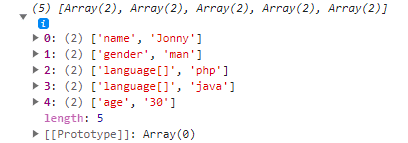
Removing a key and its value from the FormData object with key specifications
The FormData class provides the delete method, which allows you to remove a key and its value from the object. You can delete a key and its value using delete(‘key’).
document.querySelector('form').addEventListener('submit', function(e){
const formData = new FormData(this)
formData.delete('name') // delete method allows you to delete key and value from the FormData object
console.log(formData)
e.preventDefault()
})
delete method allows you to delete key and value from the FormData object
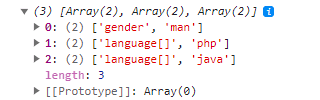
Looping through the FormData object.
If you want to perform repetitive processing on each key and value of the FormData object, you can achieve this using forEach. Although entries(), for … of, and for … in can also be used for looping, personally, I find forEach to be neater.
document.querySelector('form').addEventListener('submit', function(e){
const formData = new FormData(this)
formData.forEach((val, key) => {
console.log(key +':'+ val)
})
e.preventDefault()
})
You can loop through the FormData object using forEach
.

Sending the FormData object to the server-side.
Let’s send the FormData object to the server-side and verify if the form contents are being properly sent.
In this case, we are using PHP as the server-side language. The code on the server-side is simple, just encodes the received data as JSON and returns it.
<?php
echo json_encode($_POST);
On the JavaScript side, we will use fetch to send the FormData object to the server-side and then verify the returned value.
document.querySelector('form').addEventListener('submit', function(e){
const formData = new FormData(this)
fetch('post.php', { method: "POST", body: formData })
.then((response)=> response.json())
.then((data)=>{
console.log(data)
})
e.preventDefault()
})
Confirmed that the form content is being sent successfully!

That is all, it was about how to get all form values at once and send them using FormData class!