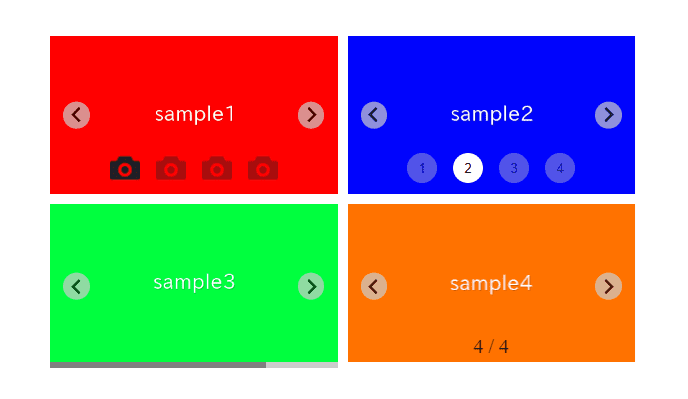
There may be times when you want to customize the pagination of a Splide slider.
In this article, we will describe how to change the color, position, size, and image of pagination, as well as adding numbers to dots, using fraction notation, or converting it into a progress bar.
We will proceed on the assumption that you know how to create a regular slider in Splide.js, so if you are new to Splide.js, please refer here first.
[Splide] How to create a simple slider with Splide.js
Table of Contents
- Create a regular Splide slider
- How to change the color of pagination
- How to change the position of pagination
- How to change the size of pagination
- How to replace pagination with image
How to add numbers to the pagination dots.- How to convert pagination to fraction notation
- How to conert the pagination to progressbar
Create a regular Splide slider
For clarity, let’s start by creating a regular Splide slider and then proceed to make modifications from there.
<link href="https://cdn.jsdelivr.net/npm/@splidejs/splide@4/dist/css/splide.min.css" rel="stylesheet">
<script src="https://cdn.jsdelivr.net/npm/@splidejs/splide@4/dist/js/splide.min.js"></script>
<style>
.sample-slider {
width: 70%;
margin: 0 auto;
}
.sample-slider img {
width: 100%;
}
</style>
<div class="splide sample-slider">
<div class="splide__track">
<ul class="splide__list">
<li class="splide__slide"><img src="./img/sample1.png"></li>
<li class="splide__slide"><img src="./img/sample2.png"></li>
<li class="splide__slide"><img src="./img/sample3.png"></li>
</ul>
</div>
</div>
<script>
new Splide('.sample-slider', {
type : 'loop',
}).mount()
</script>
Now we can see the regular Splide slider.
How to change the color of pagination
In Splide.js, you can specify the color of pagination using the splide__pagination__page class.
Since opacity: 0.7 is set to all pagination elements, we need to disable transparency only for the active pagination.
Additionally, increasing the opacity of inactive pagination elements can improve visibility.
.sample-slider .splide__pagination__page {
background: yellow; /* Specify the pagination color */
opacity: .4; /* Increase the opacity of inactive pagination */
}
.sample-slider .splide__pagination__page.is-active {
opacity: 1; /* Disable transparency of the active pagination */
}
The pagination color has been changed.
How to change the position of pagination
You can adjust the position of the pagination using the splide__pagination class.
Since bottom: 0.5em is set by default, so specifying a smaller value, for example, will move the pagination downwards.
.sample-slider .splide__pagination {
bottom: -1em;
}
The pagination position has been changed.
Additionally, you can initialize the bottom property and specify the position from the top using the top property.
.sample-slider .splide__pagination {
bottom: initial;
top: 1em;
}
How to change the size of pagination
You can specify the size of the pagination using the width and height properties in the splide__pagination__page class.
If you want to create space between the dots, you can use the gap property in the splide__pagination class.
Additionally, in Splide.js, the size of the active pagination is larger by default. You can unify the sizes by adding transform: scale(1).
.sample-slider .splide__pagination {
gap: 10px; /* Space between the dots */
}
.sample-slider .splide__pagination__page {
width: 30px; /* Width of pagination dots */
height: 30px; /* Height of pagination dots */
}
.sample-slider .splide__pagination__page.is-active {
transform: scale(1); /* Unify the size of pagination dots */
}
The pagination size has been changed.
How to replace pagination with image
There may be times when you want to replace the pagination with custom images.
By initializing the border-radius and background properties and specifying the path of the image using background-image property, you can replace pagination with images.
.sample-slider .splide__pagination__page {
border-radius: initial;
background: initial;
background-size: 100%;
background-image: url(./img/pagination_icon.png);
}
The paginaion has been replaced with image.
How to add numbers to the pagination dots.
As one of the customization patterns for pagination, there’s an design which numbers are included within the dots.
We recently discovered that this can actually be achieved using only CSS.
.sample-slider .splide__pagination {
counter-reset: pagination-num;
}
.sample-slider .splide__pagination__page:before {
counter-increment: pagination-num;
content: counter( pagination-num );
}
We have been able to put numbers inside the pagination dots.
How to convert pagination to fraction notation
There might be times when you want to create pagination where the current position and the total number of slides are represented in fraction notation.
Unfortunately, it seems that there is no built-in option in Splide.js to display pagination in fraction notation (as of March 2024).
However, don’t worry, you can achieve this using event handlers.
const sample_slider = new Splide('.sample-slider', {
type : 'loop',
})
sample_slider.on('mounted move', function(){
const s = sample_slider
const pagination = s.root.querySelector('.splide__pagination')
pagination.innerHTML = `<span>${s.index + 1 + ' / ' + s.length}</span>`
})
sample_slider.mount()
The size can be specified with the font-size property of the splide__pagination class.
.sample-slider .splide__pagination {
font-size: 20px;
}
Pagination has been fraction notation.
How to conert the pagination to progressbar
Finally, here’s how to convert the pagination to a progress bar.
This requires additional code to be added to HTML, CSS, and JavaScript.
In HTML, place the frame of the progress bar.
<div class="splide sample-slider">
<div class="splide__track">
<ul class="splide__list">
<li class="splide__slide"><img src="./img/sample1.png"></li>
<li class="splide__slide"><img src="./img/sample2.png"></li>
<li class="splide__slide"><img src="./img/sample3.png"></li>
</ul>
</div>
<!------ ↓ added ↓ ------>
<div class="progressbar">
<div class="bar"></div>
</div>
<!------ ↑ added ↑ ------>
</div>
In CSS, specify the color, height, and movement speed.
.sample-slider .progressbar {
background: #ccc;
}
.sample-slider .progressbar .bar {
background: greenyellow;
transition: 400ms ease;
height: 6px;
}
In JavaScript, calculate the progress rate and move the bar accordingly.
Also, make sure to hide the default pagination.
const sample_slider = new Splide('.sample-slider', {
type : 'loop',
pagination: false,
})
sample_slider.on('mounted move', function(){
const s = sample_slider
const bar = s.root.querySelector('.progressbar .bar')
bar.style.width = (s.index + 1) / s.length * 100 + '%'
})
sample_slider.mount()
Pagination has been into a progress bar.
That is all, it was about how to customize pagination of Splide slider.