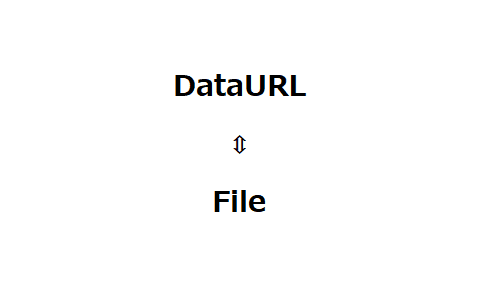
In this article, I am going to describe about how to convert DataURL to File and how to convert File to DataURL.
Table of Contents
DataURL to File
Conversion from DataURL to File is possible by combining fetch method and File Api.
DataURL is once converted to Blob By fetch method and then converted to File by File Api.
You can do any operation againt the converted File in the then method.
const dataUrl = "data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAAAEAAAABCAYAAAAfFcSJAAAAAXNSR0IArs4c6QAAAA1JREFUGFdjOHLkyH8AB+gDTOQq89IAAAAASUVORK5CYII="
fetch(dataUrl)
.then(response => response.blob())
.then(blob => {
const file = new File([blob], "sample.png", {type: blob.type})
console.log(file) //File object
})
DataURL to File as a function
In the way which I wrote above, we have to write the continuation in the then method.
By getting together the conversion process as a function as below, we can save the converted File object into a variable.
;(async function()
//some code
//some code
const dataUrl = "data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAAAEAAAABCAYAAAAfFcSJAAAAAXNSR0IArs4c6QAAAA1JREFUGFdjOHLkyH8AB+gDTOQq89IAAAAASUVORK5CYII="
const file = await url2File(dataUrl, 'smaple.png')
//some code
//some code
})()
async function url2File(url, fileName){
const blob = await (await fetch(url)).blob()
return new File([blob], fileName, {type: blob.type})
}
[Javascript]How to save a fetch result into a variable
File to DataURL
FileReader might be helpful to convert File to DataURL.
You can do any operation againt the returned DataURL in the onload event.
const file = new File(["hoge"], "sample.png", {type: 'image/png'})
const reader = new FileReader()
reader.readAsDataURL(file)
reader.onload = function(){
const dataUrl = reader.result
console.log(dataUrl) //DataURL
}
File to DataURL as a function
In the way which I wrote above, we have to write the continuation in the onload event.
By getting together conversion process as a function as below, we can save the converted DataURL into a variable.
;(async function(){
//some code
//some code
const file = new File(["hoge"], "sample.png", {type: 'image/png'})
const dataUrl = await convert2DataUrl(file)
//some code
//some code
})()
async function convert2DataUrl(blobOrFile){
const reader = new FileReader()
reader.readAsDataURL(blobOrFile)
await new Promise(resolve => reader.onload = function(){ resolve() })
return reader.result
}
Though Promise is also used and little bit complecated, details are explained in the below article.
[Javascript]How to save FileReader result into a variable
That is all, it was about how to convert DataURL to File and how to convert File to DataURL.