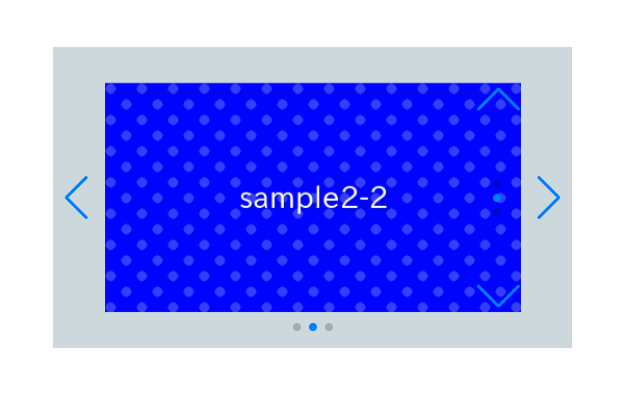
In this article, we will provide a guide, along with a demo, on how to create nested sliders using Swiper.js and highlight the important considerations for implementing them.
I’m going to pursue the matter on the premise that you know how to make normal slider with Swiper.js. So if you are new to Swiper.js, firstly check the below article.
[Swiper] How to create a simple slider with swiper.js
Table of Contents
Nested slider with horizontal child sliders
Creating a nested slider with horizontal child sliders is straightforward. All you need to do is prepare additional sliders inside each swiper-slide element. The following example demonstrates a nested slider with a total of 9 slides in a 3×3 grid.
Note: There is one important consideration which is highlighted in a blue box below the demo.
<!DOCTYPE html>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/swiper@8/swiper-bundle.min.css">
<script src="https://cdn.jsdelivr.net/npm/swiper@8/swiper-bundle.min.js"></script>
<style>
.swiper img{
width: 100%;
}
.nested-slider-h {
background-color: #CFD8DC;
width: 50vw;
padding: 40px 0!important;
}
.nested-slider-h .nested-slider-child{
width: 80%;
}
</style>
<div class="swiper nested-slider-h">
<div class="swiper-wrapper">
<div class="swiper-slide">
<div class="swiper nested-slider-child">
<div class="swiper-wrapper">
<div class="swiper-slide"><img src="./img/sample1-1.png"></div>
<div class="swiper-slide"><img src="./img/sample1-2.png"></div>
<div class="swiper-slide"><img src="./img/sample1-3.png"></div>
</div>
<div class="swiper-pagination"></div>
<div class="swiper-button-prev child-navi-prev"></div>
<div class="swiper-button-next child-navi-next"></div>
</div>
</div>
<div class="swiper-slide">
<div class="swiper nested-slider-child">
<div class="swiper-wrapper">
<div class="swiper-slide"><img src="./img/sample2-1.png"></div>
<div class="swiper-slide"><img src="./img/sample2-2.png"></div>
<div class="swiper-slide"><img src="./img/sample2-3.png"></div>
</div>
<div class="swiper-pagination"></div>
<div class="swiper-button-prev child-navi-prev"></div>
<div class="swiper-button-next child-navi-next"></div>
</div>
</div>
<div class="swiper-slide">
<div class="swiper nested-slider-child">
<div class="swiper-wrapper">
<div class="swiper-slide"><img src="./img/sample3-1.png"></div>
<div class="swiper-slide"><img src="./img/sample3-2.png"></div>
<div class="swiper-slide"><img src="./img/sample3-3.png"></div>
</div>
<div class="swiper-pagination"></div>
<div class="swiper-button-prev child-navi-prev"></div>
<div class="swiper-button-next child-navi-next"></div>
</div>
</div>
</div>
<div class="swiper-pagination"></div>
<div class="swiper-button-prev parent-navi-prev"></div>
<div class="swiper-button-next parent-navi-next"></div>
</div>
<script>
new Swiper('.nested-slider-h', {
loop: true,
pagination: {
el: '.swiper-pagination',
clickable: true,
},
navigation: {
nextEl: ".parent-navi-next",
prevEl: ".parent-navi-prev",
},
})
new Swiper('.nested-slider-h .nested-slider-child', {
loop: true,
nested: true,
pagination: {
el: '.swiper-pagination',
clickable: true,
},
navigation: {
nextEl: ".child-navi-next",
prevEl: ".child-navi-prev",
},
})
</script>
Now we can a nested slider with horizontal child sliders!
When using the same class for the navigation of the parent and child sliders, the behavior can become problematic. Clicking the navigation of the child slider will cause both the parent and child sliders to move together. Therefore, it is necessary to use different class names for each.
In the above example, we have retained the default swiper-button-next/prev classes for visual purposes. However, we have added the classes parent-navi-next/prev to the navigation elements of the parent slider, and child-navi-next/prev to the navigation elements of the child sliders.
It is also important to specify nested: true to allow the child sliders to be moved by dragging.
Nested slider with vertical child sliders
To create a nested slider with vertical child sliders, you need to make a few modifications to the previous code.
・Change the class of the parent slider from nested-slider-h to nested-slider-v (any class name ok, just to not conflict with other elements).
・Add direction: “vertical” to the Swiper options of the child sliders.
・Add some additional styles.
<!DOCTYPE html>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/swiper@8/swiper-bundle.min.css">
<script src="https://cdn.jsdelivr.net/npm/swiper@8/swiper-bundle.min.js"></script>
<style>
.swiper img{
width: 100%;
}
.nested-slider-v {
background-color: #CFD8DC;
width: 50vw;
height: 29vw;
}
.nested-slider-v .swiper-slide {
display: flex;
align-items: center;
}
.nested-slider-v .nested-slider-child [class^=swiper-button]{
transform:rotate(90deg);
top: initial;
left: initial;
right: 10px;
margin: 0;
}
.nested-slider-v .nested-slider-child .swiper-button-prev{
top:0;
}
.nested-slider-v .nested-slider-child .swiper-button-next{
bottom:0;
}
.nested-slider-v .nested-slider-child .swiper-pagination{
right:20px;
}
.nested-slider-v .nested-slider-child{
width: 80%;
height: 80%;
}
</style>
<div class="swiper nested-slider-v">
<div class="swiper-wrapper">
<div class="swiper-slide">
<div class="swiper nested-slider-child">
<div class="swiper-wrapper">
<div class="swiper-slide"><img src="./img/sample1-1.png"></div>
<div class="swiper-slide"><img src="./img/sample1-2.png"></div>
<div class="swiper-slide"><img src="./img/sample1-3.png"></div>
</div>
<div class="swiper-pagination"></div>
<div class="swiper-button-prev child-navi-prev"></div>
<div class="swiper-button-next child-navi-next"></div>
</div>
</div>
<div class="swiper-slide">
<div class="swiper nested-slider-child">
<div class="swiper-wrapper">
<div class="swiper-slide"><img src="./img/sample2-1.png"></div>
<div class="swiper-slide"><img src="./img/sample2-2.png"></div>
<div class="swiper-slide"><img src="./img/sample2-3.png"></div>
</div>
<div class="swiper-pagination"></div>
<div class="swiper-button-prev child-navi-prev"></div>
<div class="swiper-button-next child-navi-next"></div>
</div>
</div>
<div class="swiper-slide">
<div class="swiper nested-slider-child">
<div class="swiper-wrapper">
<div class="swiper-slide"><img src="./img/sample3-1.png"></div>
<div class="swiper-slide"><img src="./img/sample3-2.png"></div>
<div class="swiper-slide"><img src="./img/sample3-3.png"></div>
</div>
<div class="swiper-pagination"></div>
<div class="swiper-button-prev child-navi-prev"></div>
<div class="swiper-button-next child-navi-next"></div>
</div>
</div>
</div>
<div class="swiper-pagination"></div>
<div class="swiper-button-prev parent-navi-prev"></div>
<div class="swiper-button-next parent-navi-next"></div>
</div>
<script>
new Swiper('.nested-slider-v', {
loop: true,
pagination: {
el: '.swiper-pagination',
clickable: true,
},
navigation: {
nextEl: ".parent-navi-next",
prevEl: ".parent-navi-prev",
},
})
new Swiper('.nested-slider-v .nested-slider-child', {
loop: true,
direction: "vertical",
pagination: {
el: '.swiper-pagination',
clickable: true,
},
navigation: {
nextEl: ".child-navi-next",
prevEl: ".child-navi-prev",
},
})
</script>
Now we can a nested slider with vertical child sliders!
That is all. It was about how to create nested slider using Swiper.js