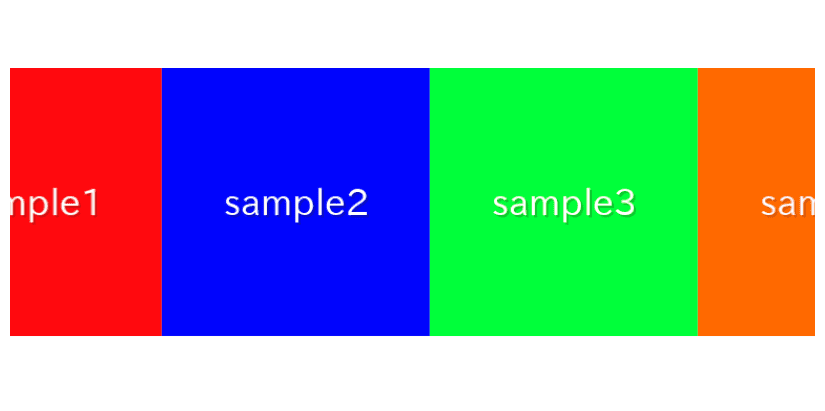
In this article, we are going to describe how to make infinite loop slider which continue to flow automatically using swiper.js and React.
We will proceed with the understanding that you are familiar with the basic usage of Swiper.js and React.
If you are new to creating a Swiper slider in React, you might find this resource helpful as a starting point.
How to use Swiper.js with React
Table of Contents
①Make a slider which does not have arrows and dots
We will be using Swiper 8.
The reason for this choice is that Swiper 9 and 10 do not work well when we use the loop option and slidesPerView option together.
npm i swiper@8
Arrows and dots are unnecessary because we are going to make a slider which continue to flow automatically.
Set loop option true.
import { Swiper, SwiperSlide } from 'swiper/react'
import 'swiper/css'
import './style.css'
export default function SampleSlider() {
return (
<Swiper
className="sample-slider"
loop={true}
>
<SwiperSlide><img src="/img/sample1.png" /></SwiperSlide>
<SwiperSlide><img src="/img/sample2.png" /></SwiperSlide>
<SwiperSlide><img src="/img/sample3.png" /></SwiperSlide>
<SwiperSlide><img src="/img/sample4.png" /></SwiperSlide>
</Swiper>
)
}
.sample-slider {
width: 70%;
}
.sample-slider img {
width: 100%;
}
Now we can see a slider which does not have arrows and dots.
You can move the slide with swiping.
②Make the slides autoplay
We can make the slides autoplay by autoplay option.
To enable autoplay option, you need to import the module.
Set delay option 0 to move slide automatically without stopping.
Add three lines as follows:
import { Swiper, SwiperSlide } from 'swiper/react'
import { Autoplay } from 'swiper' // added
import 'swiper/css'
import './style.css'
export default function SampleSlider() {
return (
<Swiper
className="sample-slider"
modules={[Autoplay]} // added
autoplay={{delay:0}} // added
loop={true}
>
<SwiperSlide><img src="/img/sample1.png" /></SwiperSlide>
<SwiperSlide><img src="/img/sample2.png" /></SwiperSlide>
<SwiperSlide><img src="/img/sample3.png" /></SwiperSlide>
<SwiperSlide><img src="/img/sample4.png" /></SwiperSlide>
</Swiper>
)
}
Autoplay slider has been created.
③Change slide speed and number of shown items
We can change slide speed by speed option, and number of shown items by slidesPerView option.
import { Swiper, SwiperSlide } from 'swiper/react'
import { Autoplay } from 'swiper'
import 'swiper/css'
import './style.css'
export default function SampleSlider() {
return (
<Swiper
className="sample-slider"
modules={[Autoplay]}
loop={true}
autoplay={{delay:0}}
slidesPerView={3} // added
speed={3000} // added
>
<SwiperSlide><img src="/img/sample1.png" /></SwiperSlide>
<SwiperSlide><img src="/img/sample2.png" /></SwiperSlide>
<SwiperSlide><img src="/img/sample3.png" /></SwiperSlide>
<SwiperSlide><img src="/img/sample4.png" /></SwiperSlide>
</Swiper>
)
}
④Smooth the slide motion.
We can smooth the motion of slide by adding CSS as below.
.sample-slider .swiper-wrapper{
transition-timing-function: linear;
}
Infinite loop slider has been created!
⑤Stop autoplay when hovering
Some visitors to your website perhaps sometimes want to stop the autoplay slider and concentrate
a specific slide.
For such visitors, lets make our infinite loop slider more better.
By adding pauseOnMouseEnter: true, you can pause the autoplay when hovering.
By adding disableOnInteraction: false, you can resume autoplay when the hover is removed.
autoplay={{
delay:0,
pauseOnMouseEnter: true, // added
disableOnInteraction: false, // added
}}
The autoplay would stop when hovering, and would restart when the hover is removed.
It would not stop instantly but stop at the end of slide switching.
⑥Reverse the autoplay direction
We can reverse the autoplay direction by adding reverseDirection: true into autoplay option.
autoplay={{
delay:0,
pauseOnMouseEnter: true,
disableOnInteraction: false,
reverseDirection: true, // added
}}
The autoplay direction has reversed.
The full text of source code
In the end, I put the full text of source code.
npm i swiper@8
import { Swiper, SwiperSlide } from 'swiper/react'
import { Autoplay } from 'swiper'
import 'swiper/css'
import './style.css'
export default function SampleSlider() {
return (
<Swiper
className="sample-slider"
modules={[Autoplay]}
loop={true}
autoplay={{
delay:0,
pauseOnMouseEnter: true, // stop autoplay when hovering
disableOnInteraction: false, // restart autoplay when hover is removed
reverseDirection: true, // reverse the autoplay direction
}}
slidesPerView={3}
speed={3000}
>
<SwiperSlide><img src="/img/sample1.png" /></SwiperSlide>
<SwiperSlide><img src="/img/sample2.png" /></SwiperSlide>
<SwiperSlide><img src="/img/sample3.png" /></SwiperSlide>
<SwiperSlide><img src="/img/sample4.png" /></SwiperSlide>
</Swiper>
)
}
.sample-slider {
width: 70%;
}
.sample-slider img {
width: 100%;
}
.sample-slider .swiper-wrapper{
transition-timing-function: linear;
}
That is all, it was about how to make infinite loop slider using swiper.js and React.