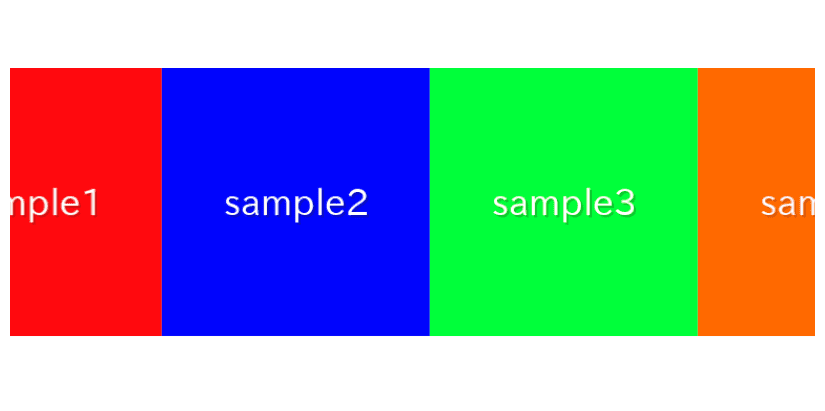
In this article, we are going to describe how to make infinite loop slider which continue to flow automatically using swiper.js.
We are going to pursue the matter on the premise that you know how to make normal slider with Swiper.js. So if you are new to Swiper.js, firstly check the below article.
[Swiper] How to create a simple slider with swiper.js
Table of Contents
①Make a slider without arrows and dots
Arrows and dots are unnecessary because we are going to make a slider which continue to flow automatically.
Set loop option true.
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/swiper@8/swiper-bundle.min.css">
<script src="https://cdn.jsdelivr.net/npm/swiper@8/swiper-bundle.min.js"></script>
<style>
.sample-slider{
width:70%;
}
.sample-slider img{
width: 100%;
}
</style>
<div class="swiper sample-slider">
<div class="swiper-wrapper">
<div class="swiper-slide"><img src="./img/sample1.png"></div>
<div class="swiper-slide"><img src="./img/sample2.png"></div>
<div class="swiper-slide"><img src="./img/sample3.png"></div>
<div class="swiper-slide"><img src="./img/sample4.png"></div>
</div>
</div>
<script>
const swiper = new Swiper('.sample-slider', {
loop: true,
})
</script>
Now we can see a slider which does not have arrows and dots.
You can move the slide with swiping.
②Make the slides autoplay
Make the slides autoplay by autoplay option.
Set delay option 0 to move slide automatically without stopping.
const swiper = new Swiper('.sample-slider', {
loop: true,
autoplay: { //add
delay: 0, //add
}, //add
})
Autoplay slider has been created.
③Change slide speed and number of shown items
We can change slide speed by speed option, and number of shown items by slidesPerView option.
const swiper = new Swiper('.sample-slider', {
loop: true,
autoplay: {
delay: 0,
},
speed: 3000, //add
slidesPerView: 3, //add
})
④Smooth motion of slide
Smooth motion of slide by adding CSS as below.
.sample-slider .swiper-wrapper{
transition-timing-function: linear;
}
Infinite loop slider has been created!
⑤Stop the autoplay when hovering
Some visitors to your website perhaps sometimes want to stop the autoplay slider and concentrate
a specific slide.
For such visitors, lets make our infinite loop slider more better.
By adding pauseOnMouseEnter: true, you can pause the autoplay when hovering.
By adding disableOnInteraction: false, you can resume autoplay when the hover is removed.
const swiper = new Swiper('.sample-slider', {
loop: true,
autoplay: {
delay: 0,
pauseOnMouseEnter: true, // added
disableOnInteraction: false, // added
},
speed: 3000,
slidesPerView: 3,
})
The autoplay would stop when hovering, and would restart when the hover is removed.
It would not stop instantly but stop at the end of slide switching.
⑥Reverse the autoplay direction
We can reverse the autoplay direction by adding reverseDirection: true into autoplay option.
const swiper = new Swiper('.sample-slider', {
loop: true,
autoplay: {
delay: 0,
pauseOnMouseEnter: true,
disableOnInteraction: false,
reverseDirection: true, // added
},
speed: 3000,
slidesPerView: 3,
})
The autoplay direction has reversed.
About loop not working
We noticed that some of the web developers were discussing issues with a loop not functioning properly.
We have investigated the causes and solutions and compiled them into a separate article.
If you are experiencing problems with the loop not working correctly or stopping midway, please refer to this article for more information.
loop with slidesPerView not working in Swiper 9 and 10
The full text of source code
In the end, I put the full text of source code.
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/swiper@8/swiper-bundle.min.css">
<script src="https://cdn.jsdelivr.net/npm/swiper@8/swiper-bundle.min.js"></script>
<style>
.sample-slider{
width:70%;
}
.sample-slider img{
width: 100%;
}
.sample-slider .swiper-wrapper{
transition-timing-function: linear;
}
</style>
<div class="swiper sample-slider">
<div class="swiper-wrapper">
<div class="swiper-slide"><img src="./img/sample1.png"></div>
<div class="swiper-slide"><img src="./img/sample2.png"></div>
<div class="swiper-slide"><img src="./img/sample3.png"></div>
<div class="swiper-slide"><img src="./img/sample4.png"></div>
</div>
</div>
<script>
const swiper = new Swiper('.sample-slider', {
loop: true,
speed: 2000,
slidesPerView: 3,
autoplay: {
delay: 0,
pauseOnMouseEnter: true, // stop autoplay when hovering
disableOnInteraction: false, // restart autoplay when hover is removed
reverseDirection: true, // reverse the autoplay direction
},
})
</script>
That is all,it is about how to make infinite loop slider with swiper.js.
Thank you very much, I have been looking for this for a while.
Muchas gracias, es el unico que me ha funcionado
Hi many thanks! I need to add this infinite loop swiper slider in wordpress. Can you give some additional details to deal with it? Have I to add this code into an index.php? Is it necessary to add it into my CSS personalized builder? Thanks!
Thank you for your question, Felix.
・Where to put the code
It depends on which page you want to display infinite loop swiper slider on.
Usually, you don’t need to modify the index.php file in WordPress.
If you are creating a static page from the WordPress admin dashboard and want to add HTML code to it, the following article can be helpful as a reference.
https://wordpress.com/support/wordpress-editor/blocks/custom-html-block/
・If necessary to add it into your CSS personalized builder
In conclusion, it is not mandatory. This source code is designed to function purely as HTML. However, from a maintainability perspective, it is better to separate styles into a CSS file.
https://www.geeksforgeeks.org/difference-between-inline-internal-and-external-css/
If you have any further questions or uncertainties, please feel free to ask!
how to stop infinite slider on hover. On hover it have to stop instantly please reply
Thanks for your question, Vivek!
I found the way which stop autoplay when hovering but not instantly.
I think we can not stop the slider until the end of switching.
I added a section which explain about how stop the autoplay when hovering in this article
⑤Stop autoplay when hovering
hi. how to use this in react component??
Thanks for your comment, yusef.
I haven’t used Swiper with React before, so I’ll do some research.
Please give me 2 or 3 days. I’ll let you know here as soon as I find out.
Hello Yusef, I apologize for the wait.
I have created an article on how to use the “infinite loop slider” in a React component.
[Swiper×React]How to make infinite loop slider
If you have any additional questions or need further clarification, please feel free to ask.
Thanks for sharing this. how can we make the slider left to right?
Thanks for your comment, Md sakib hosan!
I added a section which tells about how to make the slider left to right.
Please check it!
⑥Reverse the autoplay direction
Thank you so much!